Tags: forensics stego
Rating:
The problem description states: `"It's not LSB, its MSB! Red is Random, Green is Garbage, Blue is Boring. Hint: Only one channel is correct. Also, I like doing things top down."`
Attached is an image named lol.png:
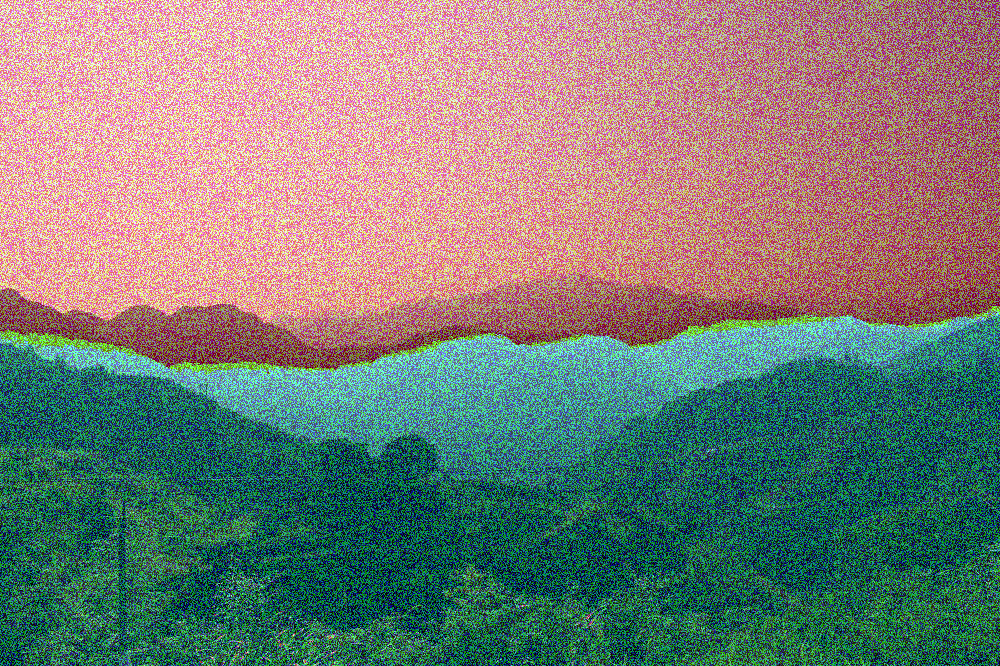
The problem's title is MSB, which stands for most signficant bit. It's the leftmost digit in the binary representation of a number. The problem statement alludes to the fact that green and red channels are useless (Green is Garbage, Red is Random). So the flag is likely found by taking the MSB of the blue pixels, concatenating them into a binary string, and finally converting it into ASCII. Since we're working with colors and RGB's values go from 0-255 (or 0000 0000-1111 1111 in binary), if the blue value of a pixel is less than 128 we appened a 1 to our binary string. Otherwise, we append a 0.

I wrote a Python script using Pillow to solve the problem:
```
from PIL import Image
# Opens up image
image = Image.open("lol.png")
loaded = image.load()
dim = image.size
imgLength = dim[0]
imgHeight = dim[1]
# Blank string that reads the binary digits
string = ""
# Goes through each pixel top-down
for x in range(imgLength):
for y in range(imgHeight):
val = loaded[x,y][2]
# This line is a bit confusing.
# I'm basically converting the number
# in the blue channel to binary.
# So 5 -> 101. Then I'm appending
# zeroes with zfill to the beginning until it reaches
# a length of 8. Finally, it takes the MSB of the binary value.
# and appends it to the binary text.
string+=((bin(val)[2:]).zfill(8))[0]
# Binary to ASCII conversion
flag = ""
while len(string) != 0:
flag+=chr(int(string[:8], 2))
string = string[8:]
print(flag)
```
This is the output:
```
pizzas, pies, and pandas. flag{MSB_really_sucks}pizzas, pies, and pandas. flag{MSB_really_sucks}pizzas, pies, and pandas. flag{MSB_really_sucks}...
```
Flag: `flag{MSB_really_sucks}`