Rating:
There is a file attached to the task: *data.txt*, which looks like
```
600 800
(255 12 0), (255 12 0), (255 12 0), (255 12 0), (255 12 0), (255 12 0)...
```
As you can see, the first line contains two numbers 600 and 800, then we see a chain of tuples. Obviously, 600 and 800 are width and height, and each of the tuple represents a pixel as (R, G, B).
Looks pretty easy! Let's write a python script to build an image from the given data (maybe this one is not quite effective, but hey, it's not ACM ICPC :) ):
```
from PIL import Image
with open('data.txt', 'r') as file:
data = file.read()
width = 600
height = 800
#There is a little hack here :)
#I simply omit the first 8 characters of data.txt, because I already know the image size
data = data[8:len(data)].split(', ')
for i in range(0,len(data)):
data[i] = data[i][1:len(data[i])-1].split(' ')
im = Image.new('RGB',(width, height))
for j in range(0,height):
for i in range(0,width):
im.putpixel((i,j), (int(data[j*width + i][0]), int(data[j*width + i][1]), int(data[j*width + i][2])))
im.save("output.png")
```
Now we have an output image:
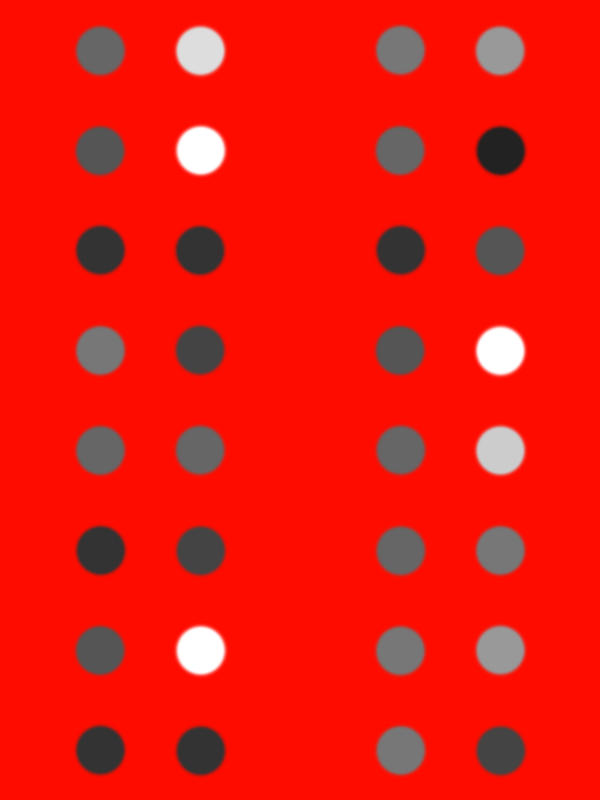
There are gray circles of different shades on this image. If open the image in any image editor (e.g. GIMP) and look at the codes of the colors of circles one by one we can see: 666666, dddddd, 777777, 999999...
It gives us the hex code: ```6d 79 5f 62 33 35 74 5f 66 6c 34 67 5f 79 33 74```
Convert it to ascii, and the flag is: ```my_b35t_fl4g_y3t```